Retrieving Reference Lists - Using the Oracle Cloud TypeScript SDK Part 7
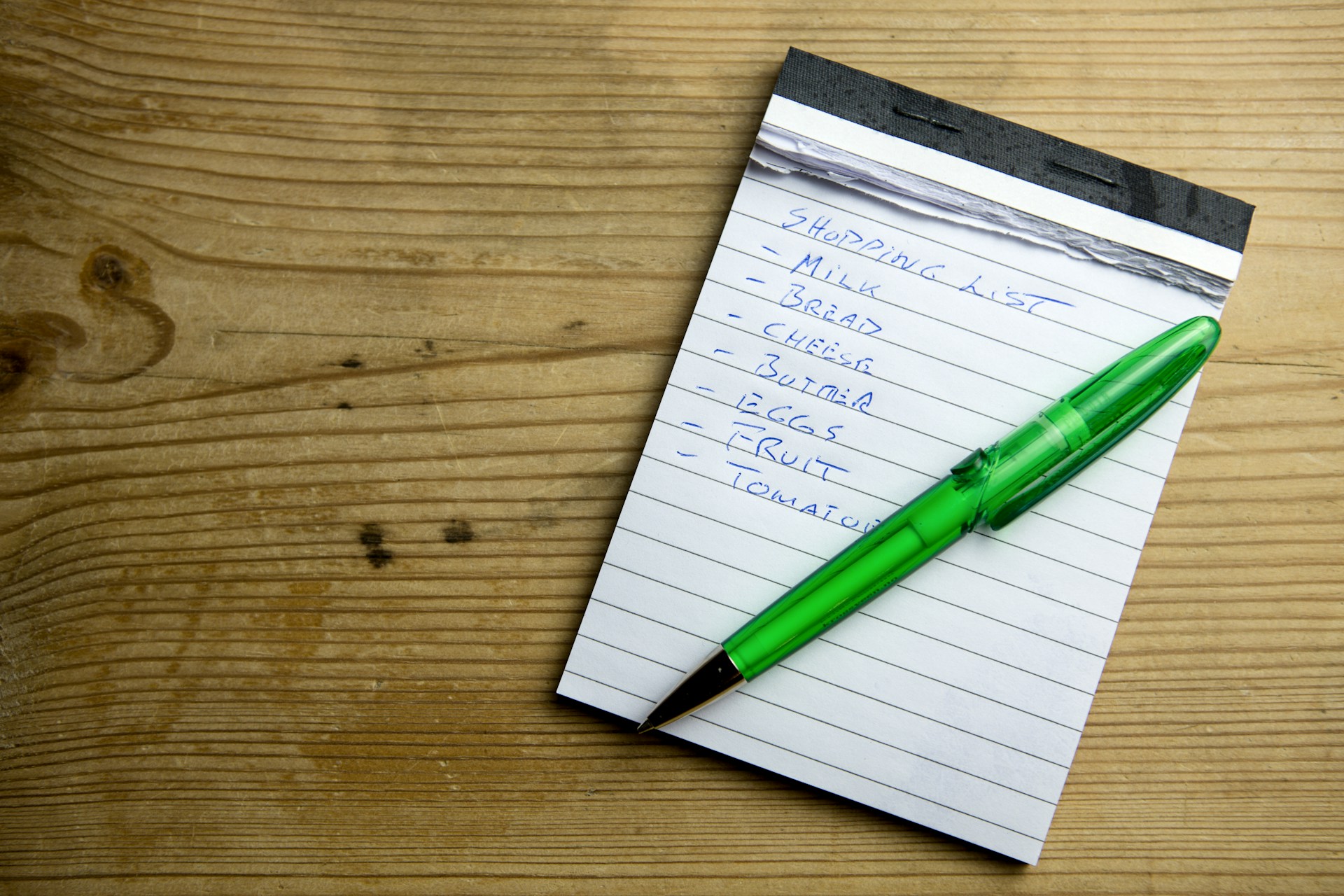
This post is the last in a series that will demonstrate how to view and manage MySQL HeatWave instances in Oracle Cloud Infrastructure (OCI). Oracle offers several SDKs that can be used to view and manage resources in OCI. In this post, we will discuss how to leverage the TypeScript/JavaScript SDK to programmatically retrieve reference data that may be needed to manage MySQL HeatWave instances using the SDK.
Prerequisites
To use the OCI SDKs, you need credentials for an OCI account with the proper permissions. While it is not necessary to install the OCI CLI, following the instructions at this post will create the same files we will need to use the SDK…with the added advantage of installing the CLI.
To follow along with this demo, you should also have Node.js installed. I am using version 21.5.0.
Creating the Node App
Before we can access the SDK, we must set up and configure it.
Initialize the Node App
Create a directory to hold your code, open a terminal/command window, and cd
into that new folder. To initialize a Node app, run the following command:
You will be prompted for information about the project. For this demo, feel free to accept all the default values.
When the init
script is complete, you should see a file named package.json
in your directory. Here is what mine looks like.
Install the Necessary Modules
Next, we will install the node modules we will need. You can install these modules using the command:
This command will install the oci-mysql
, dotenv
, and express
modules.
The oci-mysql
module contains the parts of the SDK specific to MySQL HeatWave instances. It also includes dependencies on other modules, such as oci-common
.
The bunyan
module is a JSON logging library for Node.js. I had to add this because I was getting errors while trying to run the code for this demo. I guess that there is a missing dependency somewhere. Installing bunyan
separately addressed my issues.
The dotenv
module allows us to use environment variables for information we will use in our demo.
Set up .env
In this example, we only need one environment variable, the OCID of the compartment we will use. Create a file named .env
and then add a variable named COMPARTMENT_ID
and give it the value of the compartment you want to use. It should look like the text below.
Using the SDK
Rather than break down each bit of code a little at a time, here is all the code you will need for this demo. I will break down the new pieces below.
The Code
Create a file named index.mjs
and paste in the following code.
The Breakdown
At the top of the file, we import the necessary modules, oci-common
, oci-mysl
, and dotenv
. We then call dotenv.config()
to grab the environment variables.
Next, we create a function named main()
, and inside that function, we create an instance of ConfigFileAuthenticationDetailsProvider()
called provider
. This provider reads the OCI config file created when we installed the OCI CLI. By default, the provider uses the config file located at ~/.oci/config
and the DEFAULT
config block if more than one block is specified.
Once we have an authentication provider, we need to create an instance of mysql..MysqlaasClient()
, named refClient
, and pass this provider as part of a config block.
Now that we have our refClient
, we must build the config block to specify options for our request. In this case, we only use the compartmentId
property and set the value to the COMPARTMENT_ID
environment variable.
In the next block of code, we call several SDK methods to get lists of configurations, shapes, and versions that MySQL HeatWave supports. The information returned from these requests can be used when managing MySQL HeatWave instances. For instance, when creating a MySQL HeatWave instance, we would use the name
property of a shape
to set the instance’s shape or the version
property of a specific version to set the MySQL version of the instance.
Lastly, we dump some of the information that is returned. For the configs
and shapes
, we dump the number of each and then the value of the first item in the returned array. For versions
, we dump not only the entire result (because there are only two items in the array) but also drill down to all the available versions under each versionFamily
.
Running the Code
To run the code, open a terminal/command window in the project folder and run the command:
In the console, you will see the results of the dumps from above.
Configurations
We can see that there are 107 different configurations.
Shapes
We can see there are 55 different shapes.
Versions
We can see that there are two different version families.
Version Details
We can see the different versions available in each version family.
The Wrap-Up
As shown throughout this series, we can manage MySQl HeatWave instances using the OCI SKDs. This post shows how to retrieve some of the reference data that can be used to define the properties of MySQL HeatWave instances. Be sure to check out the documentation of the TypeScript SDK to see all the available functionality.
Photo by Torbjørn Helgesen on Unsplash
Related Entries
- Listing MySQL HeatWave Instances - Using the Oracle Cloud TypeScript SDK Part 1
- Managing MySQL HeatWave Instances - Using the Oracle Cloud TypeScript SDK Part 2
- Waiters - Using the Oracle Cloud TypeScript SDK Part 3
- Listing MySQL HeatWave Backups - Using the Oracle Cloud TypeScript SDK Part 4
- Creating a MySQL HeatWave Backup - Using the Oracle Cloud TypeScript SDK Part 5